[Python] How To Access GCS Using Python With boto3
Situation
Need Access GCS Using AWS S3 SDK.
How To
Step1 : Install boto3
pip3 install boto3
Step 2 : Setting Session And Get Credential From Google Cloud Storage HMAC Key
from boto3.session import Session
from botocore.client import Config
import boto3
ACCESS_KEY = <"HMAC Key Access Key">
SECRET_KEY = <"HMAC Key Secret Key">
session = Session(aws_access_key_id=ACCESS_KEY,
aws_secret_access_key=SECRET_KEY,
region_name="ASIA-EAST1")
s3 = session.client('s3', endpoint_url='https://storage.googleapis.com',
config=Config(signature_version='s3v4'))
# or
s3 = session.resource('s3', endpoint_url='https://storage.googleapis.com',
config=Config(signature_version='s3v4'))
Step 3 Write Other Code, For Example : List Buckets & List Objects
s3r = session.resource('s3', endpoint_url='https://storage.googleapis.com',
config=Config(signature_version='s3v4'))
bucket = s3r.Bucket('gcf-sources-354846332057-asia-east1')
for f in bucket.objects.all():
print(f.key)
s3c = session.client('s3', endpoint_url='https://storage.googleapis.com',
config=Config(signature_version='s3v4'))
bucket = s3c.list_buckets()
for bucketS in bucket['Buckets']:
print(bucketS['Name'])
Finally, Code Looks Like This :
from boto3.session import Session
from botocore.client import Config
import boto3
ACCESS_KEY = <"HMAC Key Access Key">
SECRET_KEY = <"HMAC Key Secret Key">
session = Session(aws_access_key_id=ACCESS_KEY,
aws_secret_access_key=SECRET_KEY,
region_name="ASIA-EAST1")
s3r = session.resource('s3', endpoint_url='https://storage.googleapis.com',
config=Config(signature_version='s3v4'))
bucket = s3r.Bucket('<bucket name>')
for f in bucket.objects.all():
print(f.key)
s3c = session.client('s3', endpoint_url='https://storage.googleapis.com',
config=Config(signature_version='s3v4'))
bucket = s3c.list_buckets()
for bucketS in bucket['Buckets']:
print(bucketS['Name'])
Step 4 Try Run
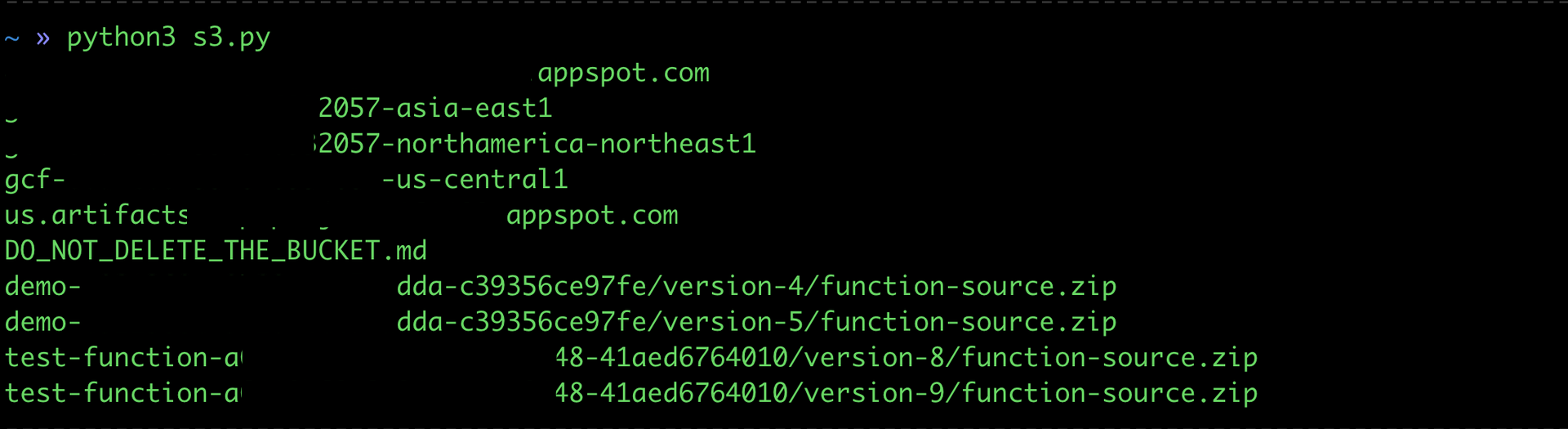